What are Data Structures?
A way to store data to use the data efficiently. Lets think about values of the data and the relationship among them, or the functions and operations that can be applied to that data.
Smart Contract Structure
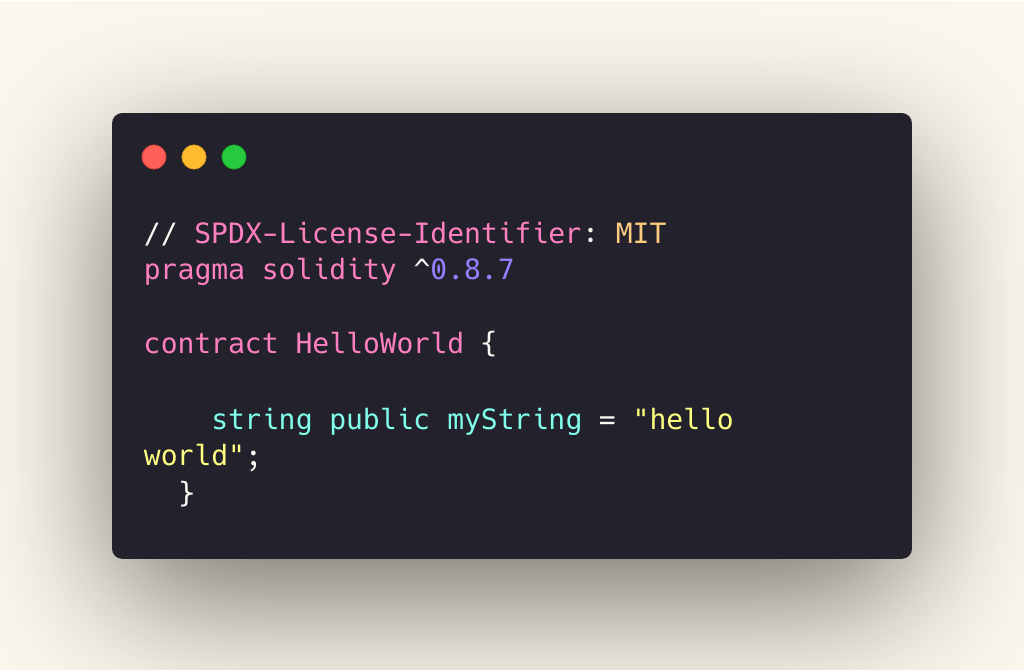
Let's look at some commonly used Data Structures in Solidity
Values and References
Data types in solidity can be classified as two types.
values
references
Values
Value means that the data stores a value. Boolean stores true or false. Int stores : -1, 0, 1.
References
References data types don't store values, instead they store references where the actual value is. Array type of type references will store where actual array elements are stored.
Signed Integer : Both positive and negative numbers.
int public i = 123;
Unsigned Integer : The number has to be greater than or equal to 0. No negative numbers.
uint public 0 = 327;
//uint = uint256 0 to 1**256-1
Booleans:
The possible value types are only true or false.
bool public a = true;
Address:
Address comes as 20 byte size to hold eth address.
address public addr = 0xAE5679C84371d53c7e2BB2870f9d00e92D506E0c;
Bytes32:
We will use it during cryptographic hash functio kechak256;
bytes32 public b32 = 0x111122223333444455556666777788889999AAAABBBBCCCCDDDDEEEEFFFFCCCC;
Arrays: Arrays are collections of variable of the same type and can be declared and used.
There are two types of Arrays.
Dynamic
Fixed Size
Dynamic Array : The size of the array can be changed.
Fixed Array : The size of the array can't be changed.
Mapping: In solidity, to store a collection of data we can either use Array or mapping. Mapping allows us to save the data/value at the key and later we can get the key. In other words, mapping is the fastest way to get any value or data.
But if we want to iterate through it, then we need to do something extra but let's get to that later.
Struct: When we need to use a bit more complicated data type with multiple properties, then we can use struct.
What is State Variable
There are three types of variable in Solidity: - State Variable - Local Variable - Global Variable State Variable : Variables that store data on the blockchain. State variables are declared inside the contract but outside the function.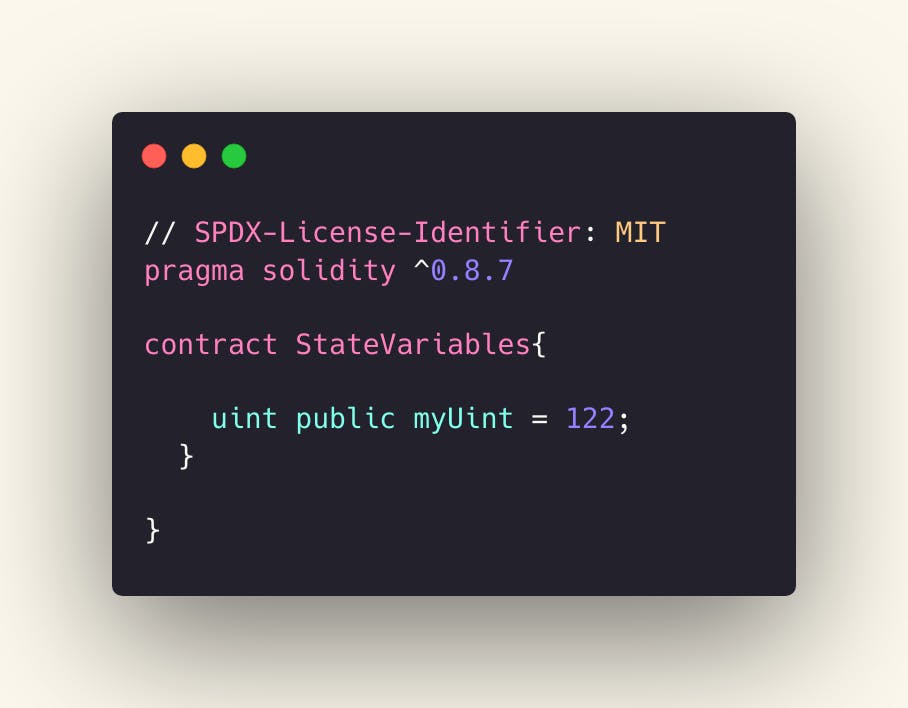
Enum :
Boolean lets you pick two choices between true and false but what if you need to pick more choices. Then enum is a perfect data type for this.
These are the basic examples of commonly used data types in solidity. It's high level detailed explanation.